Have you ever wondered how Node.js, renowned for its asynchronous prowess, handles resource-intensive tasks while maintaining responsiveness?
Enter worker threads, a powerful concept that empowers Node.js applications to juggle heavy calculations and CPU-bound operations with finesse. In this blog, we delve into the world of worker threads, unraveling their significance, inner workings, benefits, drawbacks, and practical applications.
Understanding the Node.js Landscape
To grasp the role of worker threads, it’s essential to first comprehend the architecture of Node.js. When a Node.js process comes to life, it operates within the following parameters:
- One process
- One thread
- One event loop
- One JS Engine Instance
- One Node.js Instance
In simpler terms, Node.js operates on a single thread, allowing only one process to occur within the event loop at any given time. This design simplifies JavaScript usage by circumventing concurrency complications.
The Challenge of CPU-Intensive Tasks
Yet, like any technology, Node.js has its limitations. For instance, CPU-intensive operations, such as intricate calculations or memory-intensive tasks, can obstruct the seamless flow of other processes. Similarly, a server grappling with CPU-intensive code can inadvertently stall the event loop, causing delays in handling incoming requests. Here, the distinction between “blocking” and “non-blocking” functions becomes paramount. Blocking functions hinder the event loop’s progress, while non-blocking functions permit concurrent execution.
Differentiating CPU and I/O Operations
It’s crucial to discern between CPU and I/O operations. Unlike some misconceptions, Node.js code doesn’t run in parallel; rather, I/O operations, executed asynchronously, are the parallel performers.
I/O-Bound vs CPU-Bound in Node.js
- I/O-Bound: A program’s performance benefits from enhancements in resource speed. Common in Node.js apps, this scenario arises when the event loop awaits I/O operations like network, filesystem, or database tasks.
- CPU-Bound: Processing time reduces with CPU enhancements. For instance, a hashing program performs better on a faster processor. In Node.js, CPU-bound tasks can monopolize the event loop, causing delays in request handling.
The Pros of Node.js Multithreading
- Enhanced Performance: Multi-threading salvages app performance during intensive operations, preserving responsiveness and preventing crashes or freezes.
- Optimized Resource Utilization: Leveraging multi-threading, Node.js maximizes CPU core utilization, bolstering resource efficiency and scalability.
- Offloading Burden: The crux of worker threads’ utility lies in alleviating the primary thread’s strain by migrating heavy tasks, like complex calculations and image processing, to separate threads.
- Isolation and Parallelism: Worker threads ensure each thread operates independently and undisturbed. Communication between the parent thread and worker threads transpires through messaging channels.
- Improved Performance: By fragmenting CPU-intensive tasks into manageable parts, worker threads enhance application performance and fluidity.
Harnessing Worker Threads
- CPU-Intensive Tasks: Worker threads excel at handling tasks like mathematical computations, image processing, and video transcoding. By preventing main-thread blockage, they optimize performance.
- Network I/O: Concurrently manage network I/O operations, such as HTTP requests or email transmissions, enhancing main-thread multitasking.
- File I/O: Worker threads address file I/O tasks, executing extensive file operations in parallel and elevating main-thread performance.
- Scalability: Execute database queries in parallel through worker threads, enabling seamless handling of numerous requests and amplifying scalability.
- Machine Learning: Worker threads proficiently tackle machine learning tasks, like model training and data prediction, expediting processing times for large datasets.
Balancing Act: The Cons of Worker Threads
- Resource Consumption: Spawning excessive worker threads can consume memory exponentially, particularly for CPU-bound tasks. Optimal use for CPU-intensive scenarios is advised.
- Unsuitable for I/O: Worker threads are not designed for I/O operations, leading to redundancy, expenses, and compromised performance when used incorrectly.
Worker Threads’ Unique Features
- ArrayBuffers: Facilitate memory transfer between threads.
- SharedArrayBuffer: Enable memory sharing between threads for binary data.
- Atomics: Execute concurrent processes and implement conditional variables in JavaScript.
- MessagePort and MessageChannel: Support asynchronous communication and data transfer between threads.
- WorkerData: Pass startup data between threads, mimicking postMessage() behavior.
Navigating the Worker Threads API
- `const { worker, parentPort } = require(‘worker_threads’)`
- `new Worker(filename)` or `new Worker(code, { eval: true })`
- `worker.on(‘message’)` and `worker.postMessage(data)`
- `parentPort.on(‘message’)` and `parentPort.postMessage(data)`
The Promise and Precautions for Worker Threads
-
Expectations
- Passage of native handles (e.g., sockets, HTTP requests)
- Deadlock detection for enhanced thread management
- Isolation to prevent cross-thread disruptions
-
Cautions
- Worker threads do not guarantee universal performance acceleration. Consider alternatives like worker pools in specific cases.
- Avoid using worker threads for parallelizing I/O operations.
- Understand that spawning worker threads incurs a cost.
Real-World Use Cases for Thread Workers
- Image Resizing: For applications generating varied image sizes, worker threads efficiently handle CPU-intensive image resizing tasks.
- Video Compression: Thread workers prove indispensable for compressing videos in diverse sizes, crucial for streaming apps.
- File Integrity: Using thread workers, ensure cloud-stored files remain untampered by computing cryptographic hashes, bolstering security.
Conclusion
Worker threads unveil a realm of possibilities within Node.js, empowering applications to conquer CPU-intensive tasks without compromising responsiveness. Through isolation, parallelism, and strategic execution, they usher in enhanced performance and optimal resource utilization. While not a panacea, worker threads, when appropriately harnessed, elevate the Node.js experience, rendering it adaptable to diverse scenarios. By embracing both their potential and limitations, developers can seamlessly integrate worker threads to sculpt robust and dynamic applications.
Happy Coding!!!
Do feel free to Contact Us or Schedule a Call to discuss any of your projects
Author : Ms. Arusha Kamate
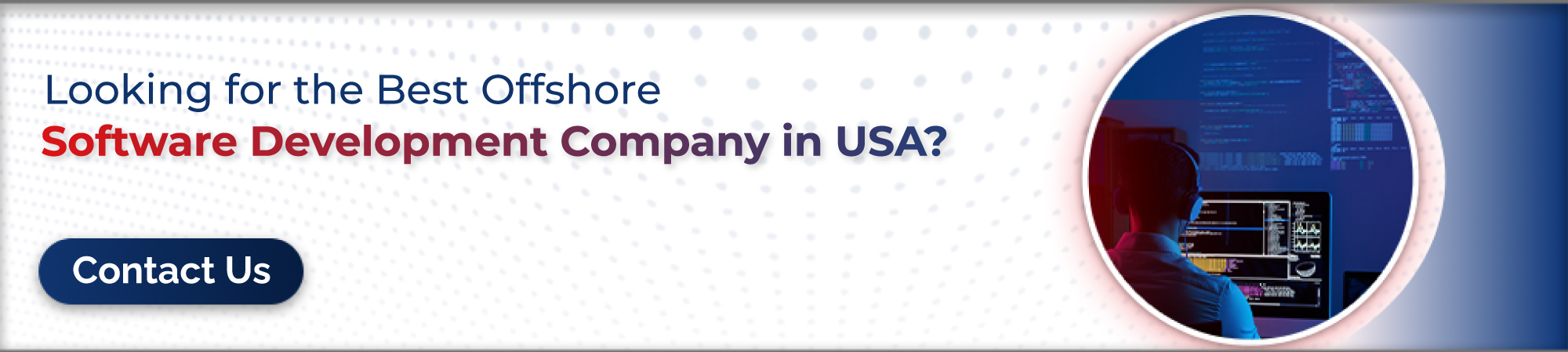